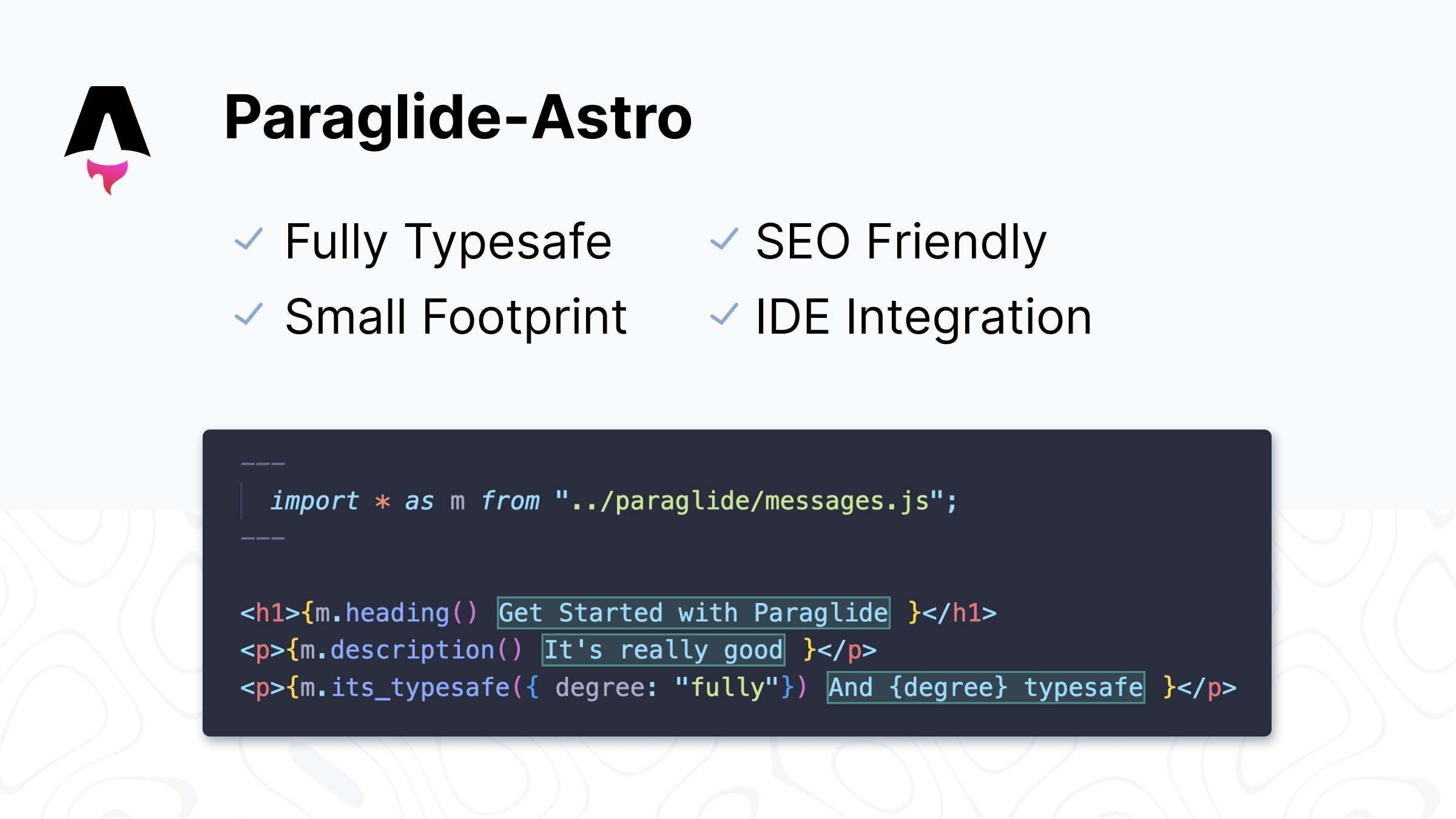
This example demonstrates how to use Paraglide JS with Astro in SSR mode. The source code can be found here.
Setup
1. If you have not initialized Paraglide JS yet, run:
2. Add the vite plugin to the astro.config.mjs
file and set output
to server
:
3. Create or add the paraglide js server middleware to the src/middleware.ts
file:
You can read more about about Astro's middleware here.
Usage
See the basics documentation for more information on how to use Paraglide's messages, parameters, and locale management.
Disabling AsyncLocalStorage in serverless environments
You can disable async local storage in serverless environments by using the disableAsyncLocalStorage
option.