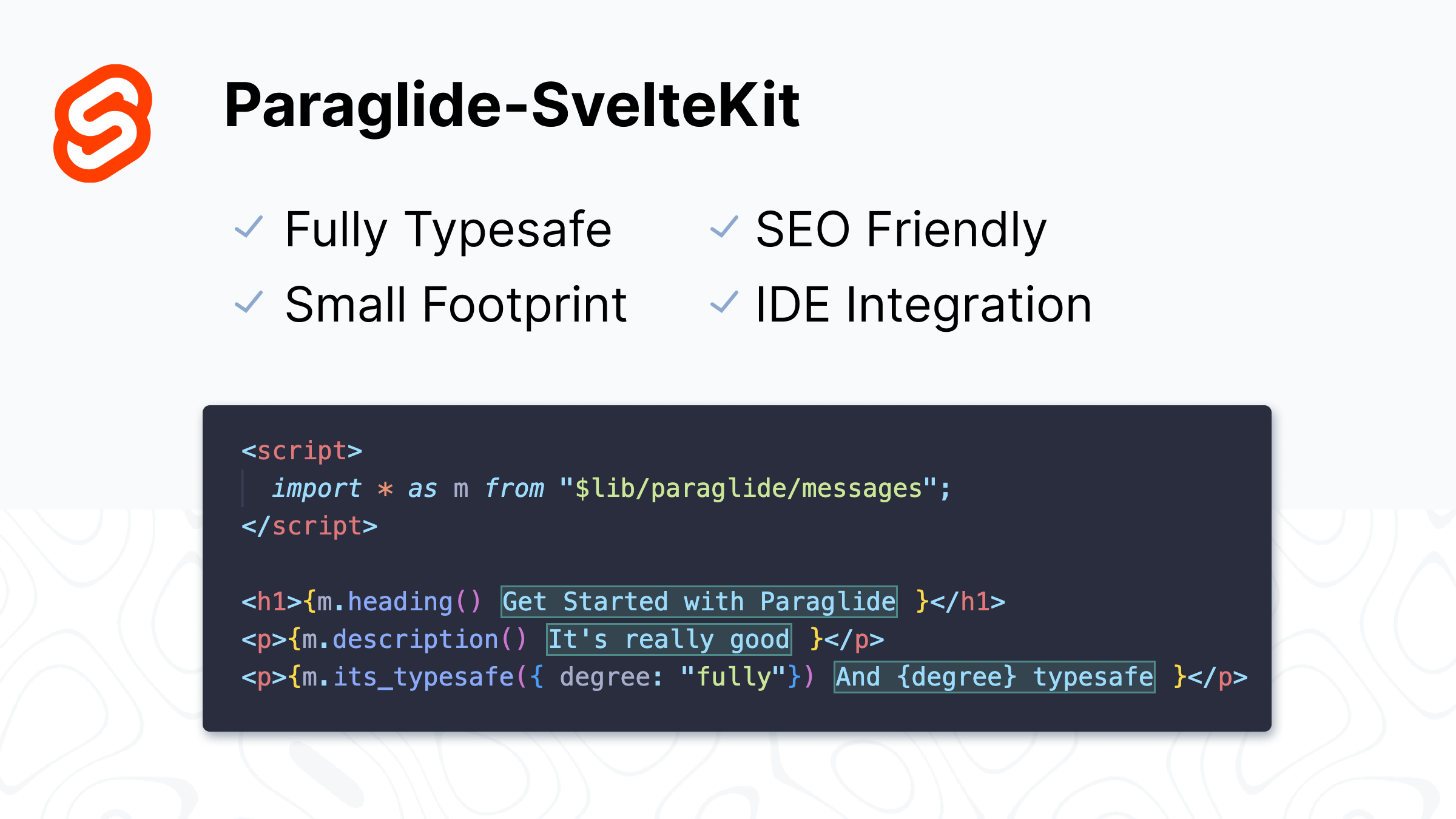
This example shows how to use Paraglide with SvelteKit.The source code can be found here.
Getting started
Install paraglide js
Add the paraglideVitePlugin()
to vite.config.js
.
Add %lang%
to src/app.html
.
See https://svelte.dev/docs/kit/accessibility#The-lang-attribute for more information.
Add the paraglideMiddleware()
to src/hooks.server.ts
Add a reroute hook in src/hooks.ts
IMPORTANT: The reroute()
function must be exported from the src/hooks.ts
file, not src/hooks.server.ts
.
Usage
See the basics documentation for more information on how to use Paraglide's messages, parameters, and locale management.
Static site generation (SSG)
Enable pre-renderering by adding the following line to routes/+layout.ts
:
Then add "invisble" anchor tags in routes/+layout.svelte
to generate all pages during build time. SvelteKit crawls the anchor tags during the build and is, thereby, able to generate all pages statically.
Troubleshooting
Disabling AsyncLocalStorage in serverless environments
If you're deploying to SvelteKit's Edge adapter like Vercel Edge or Cloudflare Pages, you can disable AsyncLocalStorage to avoid issues with Node.js dependencies not available in those environments:
No locale OR different locale when calling messages outside of .server.ts files
If you call messages on the server outside of load functions or hooks, you might run into issues with the locale not being set correctly. This can happen if you call messages outside of a request context.